If you’ve ever marveled at the capabilities of Python, a versatile and intuitive programming language, you’re not alone. Millions of coders worldwide are utilizing Python for everything from data analysis to automation. However, writing Python code is one thing; running it is another. Whether you’re a seasoned coder or an aspiring one, understanding how to run a python script is an essential skill that can broaden your horizon of opportunities. Sit tight as we take you through a journey that promises to be as enlightening as it is fascinating.
Decoding the Python Environment
First off, let’s not assume anything. Not everyone knows where to begin, especially when they have to execute python script for the first time. Python’s runtime environment is the magical place where all your coded dreams come to life. Imagine it as a stage where the actors (your code) perform a script (the program). Installing Python on your system creates this environment, allowing you to run scripts with ease.
So how do you make sure your stage is set? Start by downloading the latest Python version from its official website. Choose the appropriate installer based on your operating system. If everything’s done right, typing python –version in your command prompt or terminal should display the installed Python version.
But wait, there’s more. Once installed, your Python environment also includes something called IDLE (Integrated Development and Learning Environment), a basic editor where you can write and run python script. It’s like your personal sketchpad to quickly jot down and test out ideas.
For those looking to take things up a notch, there are dedicated Python IDEs (Integrated Development Environments) like PyCharm and Visual Studio Code that offer advanced features such as debugging and version control.
Last, but not least, ensure that Python is added to your system’s PATH environment variable. This makes sure that you can run Python scripts from any directory in your command prompt or terminal.
The Command-Line Symphony
There are countless ways to make Python dance to your tunes, but let’s start simple. One of the most straightforward methods is to run python script from command line. This approach bypasses the need for any fancy software, relying solely on your computer’s terminal or command prompt.
Open your command prompt or terminal and navigate to the directory containing your Python script. Once there, type python your_script.py and press Enter. Just like that, your Python script should execute, and you’ll see the output displayed.
However, it’s not always sunshine and rainbows. Sometimes you might encounter errors, but don’t let that dishearten you. Debugging is a part of the process and crucial for growth. Python usually provides descriptive error messages to help you understand what went wrong.
And for those who prefer a more graphical interface, remember that many IDEs offer built-in terminals where you can run python script without ever leaving the program. This feature often makes the coding process smoother and more efficient.
If you’re working on larger projects with multiple Python files, running them via the command line can help you understand how they interact with each other. You can even run scripts in the background by appending an & at the end of your command in Unix-like systems.
Bringing Scripts to Life with IDEs
While command-line methods are robust and effective, many people find comfort in the bells and whistles offered by IDEs. Integrated Development Environments act like a swiss army knife for coders, offering a range of functionalities. Most of them come with features like debugging, syntax highlighting, and even version control, thereby making it convenient for anyone wanting to know how to use python code effectively.
Among the popular IDEs, PyCharm is often hailed as the pinnacle. Its rich feature set coupled with its user-friendly interface makes it a go-to choice for professionals and hobbyists alike. Setting it up is fairly straightforward: download and install PyCharm, create a new project, and within that project, create a new Python file.
Visual Studio Code is another crowd-pleaser. Lightweight yet powerful, it’s versatile enough to handle languages other than Python. Installing the Python extension for VS Code will not only let you write Python code, but also execute python script directly from its terminal.
Jupyter Notebook is a unique IDE that focuses on data science and machine learning. Unlike other IDEs, Jupyter allows you to run individual code blocks independently, making it ideal for tasks that require iterative development and testing.
Remember, the choice of IDE often depends on personal preference and project requirements. So, take your time, experiment, and find the one that makes your coding journey more enjoyable and productive.
Finally, it’s not just about writing code; it’s also about managing it. Git integration in most IDEs lets you keep track of changes, collaborate with others, and even roll back to previous versions of your code. All of these features streamline the process of how you use python script in real-world projects.
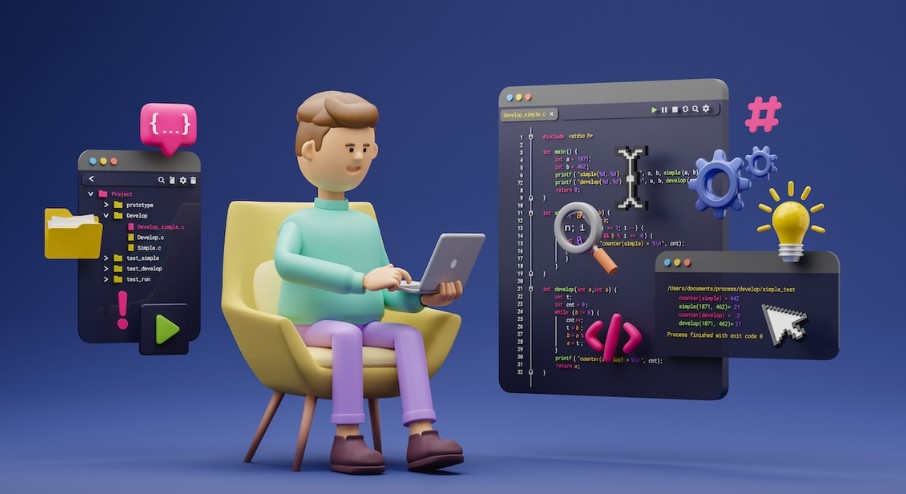
The Shebang Line: What Is It and Why It Matters
Linux and macOS users might come across something called a “shebang” line when they look into how to script with python. This line, usually the first line in your Python script, looks something like #!/usr/bin/env python3.
The shebang line specifies the interpreter for running the script and is particularly useful when you want to make your Python script executable. Instead of typing python script.py every time, you can simply make the script executable with chmod +x script.py and then run it directly using ./script.py.
So why is this useful? First, it makes your script portable. If you share it with others, they can run it without having to worry about how to run the script manually. Secondly, it saves you time and effort in the long run.
However, keep in mind that the shebang line is largely ignored on Windows systems. Windows determines the interpreter from the file extension (.py for Python) rather than looking at the shebang line.
Arguments: Talking to Your Scripts
What if you could start python code and have a two-way conversation with it? Well, you can—by using arguments. Python’s argparse library allows you to pass arguments to your script when you run it. This gives you the flexibility to customize the script’s behavior without altering the code.
For example, suppose you have a script that calculates the area of a circle. By using arguments, you could pass the radius value directly from the command line. Your script then reads this value and performs the calculation accordingly.
Arguments also open up the possibility for batch processing. Let’s say you have a Python script that renames files. By passing a list of filenames as arguments, you can rename multiple files in one go.
Moreover, arguments can act as switches or flags to activate specific sections of your code. By combining these features, you create versatile and adaptive scripts, elevating your understanding of how to use python code.
The Power of Modules and Packages
Modules and packages add a layer of versatility to how to write python script. Think of them as toolkits that contain pre-written code for a variety of tasks. Whether you want to scrape web data, manipulate images, or delve into machine learning, there’s probably a Python module that can make your life easier.
To utilize these modules, you first need to install them. The Python Package Index (PyPI) is a vast repository of modules and packages. You can install these using Python’s package manager, pip. A simple pip install package_name does the trick.
Once installed, importing a module into your script is as simple as writing import module_name. This action equips you with all the functions and variables defined in that module, enhancing your ability to create more complex and functional scripts.
By incorporating modules, you also promote code reuse and reduce redundancy. Why reinvent the wheel when you can stand on the shoulders of giants? Importing modules can significantly speed up development time and make your scripts more robust.
Finally, don’t underestimate the power of creating your own modules. If you find yourself using the same functions across multiple scripts, it might be time to bundle those functions into your own custom module.
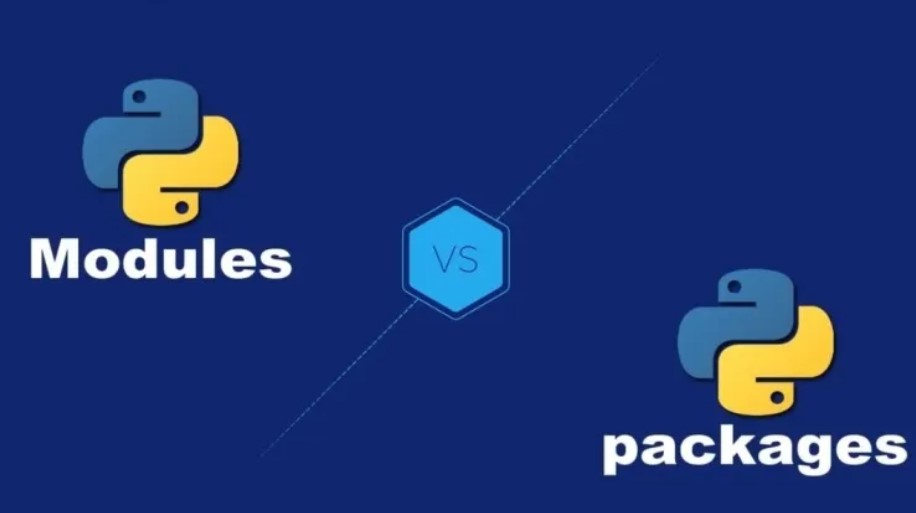
Script Automation: The Peak of Efficiency
If you’re looking to supercharge your productivity, understanding how to use python script for automation is your ticket to nirvana. Python excels in automating mundane tasks, allowing you to focus on more important matters.
You can automate almost anything: from sending emails to updating spreadsheets. Python’s smtplib library, for instance, can send emails for you. With openpyxl, you can read and write Excel spreadsheets. If you’re into web scraping, BeautifulSoup and Selenium are your best friends.
To get started with automation, identify the tasks you regularly perform. Once you’ve narrowed it down, find the relevant Python library that can perform that task. The next step is to write a script incorporating that library. Finally, you can schedule your script to run at specific times using task schedulers, like cron for Unix-based systems or Task Scheduler for Windows.
Debugging: A Necessary Evil
No matter how proficient you are at writing Python scripts, debugging is a skill you can’t afford to neglect. Error messages might look like hieroglyphics at first, but they’re actually treasure maps leading you to the issue in your code.
Python’s traceback output is particularly helpful. It shows you the series of function calls that led to the error, making it easier to locate the issue. You can also use Python’s built-in pdb debugger to step through your code one line at a time, inspecting the values of variables as you go.
IDEs come with their own debugging tools that offer advanced features like conditional breakpoints and watch expressions. These allow you to pause execution when certain conditions are met or when specific variables change, providing valuable insights into how your code is running and where it might be going wrong.
Debugging isn’t just for fixing errors; it’s also for understanding how your code is executed. By regularly debugging your scripts, you get a clearer picture of your code’s flow and logic, becoming a more proficient coder in the process.
Version Control: The Time Machine for Coders
If you’ve ever lost hours of work due to an accidental deletion or a botched update, you’ll appreciate the importance of version control. Git, a widely used version control system, can act like a time machine for your code, allowing you to go back to previous versions whenever you need.
Git integration in most IDEs makes this process even more seamless. With a few clicks, you can commit changes, create branches, and even collaborate with other developers. This is particularly useful for large projects where multiple people are working on the same codebase.
Beyond just tracking changes, Git also makes collaboration easier. By pushing your code to remote repositories like GitHub, you make it accessible to other developers. They can then clone your repository, make their own changes, and submit them for your review. This collaborative cycle is at the heart of many open-source projects and is invaluable for team-based work.
Security Measures: The Unsung Hero in Script Execution
When you’re learning how to run a python script, it’s easy to overlook one critical aspect: security. Running any script, especially one that you’ve downloaded from the internet or received from an untrusted source, poses certain risks. Understanding these risks and how to mitigate them is vital for anyone dabbling in Python scripting.
Firstly, it’s crucial to inspect the code for any malicious activity before running it. Always read through the Python script and make sure you understand what it’s doing. This is easier when dealing with shorter scripts, but for longer ones, focus on parts of the code that interact with your file system, make network requests, or execute shell commands.
Secondly, consider running the script in a virtual environment. By doing this, you can isolate the Python script from your main operating system, reducing the risk of damaging critical files. Tools like virtualenv for Python can create isolated environments that separate your project’s dependencies and settings from your system-wide settings. This is not only good for security, but also for dependency management.
Thirdly, ensure that you have updated your Python to the latest version. Developers frequently release security patches and updates, so running an older version of Python can leave you vulnerable to known security flaws. The same goes for any third-party modules or packages that your script might use. Always keep them up to date to minimize security risks.
Lastly, don’t neglect the power of permissions. Limit who can access and run your Python scripts. On Unix-like systems, you can use the chmod command to change file permissions, thereby restricting unauthorized access to your script. For example, running chmod 700 script.py would ensure that only the file’s owner can read, write, and execute the script.
By incorporating these security measures, you add an extra layer of protection, ensuring that your journey into Python scripting is not only educational, but also secure. So the next time you find yourself ready to run python script, take a moment to consider these security precautions. They might just save you from a lot of trouble.
Conclusion: Embark on a Limitless Scripting Odyssey
Congratulations, you’ve reached the end of our comprehensive guide on how to run your Python scripts. But remember, this isn’t the end of your journey; it’s just the beginning of an exciting and boundless adventure.
As you delve into the world of Python scripting, you’ll uncover the immense power and flexibility that this programming language offers. From automating everyday tasks to developing complex applications, Python’s versatility knows no bounds. Each script you write, each line of code you craft, is a step towards becoming a more proficient and creative coder.
The skills you’ve acquired here—setting up your Python environment, mastering IDEs, debugging like a pro, utilizing modules and packages, automating tasks, and understanding security measures—are the building blocks of your coding arsenal. With these tools at your disposal, you’re ready to tackle real-world challenges and bring your ideas to life.
But remember, the learning never stops. Python is a constantly evolving language, and the world of programming is always changing. So, stay curious, explore new libraries, embrace best practices, and don’t hesitate to seek help from the vibrant Python community.
So, go ahead, write those scripts, run them, debug them, and most importantly, enjoy the process. Python scripting isn’t just about lines of code; it’s about solving problems, creating solutions, and making your mark in the ever-expanding realm of technology. Embrace the limitless possibilities that Python offers, and let your scripting odyssey continue to unfold.